How To Use Filter To Only Grab Objects That Contain A Certain String From An Array
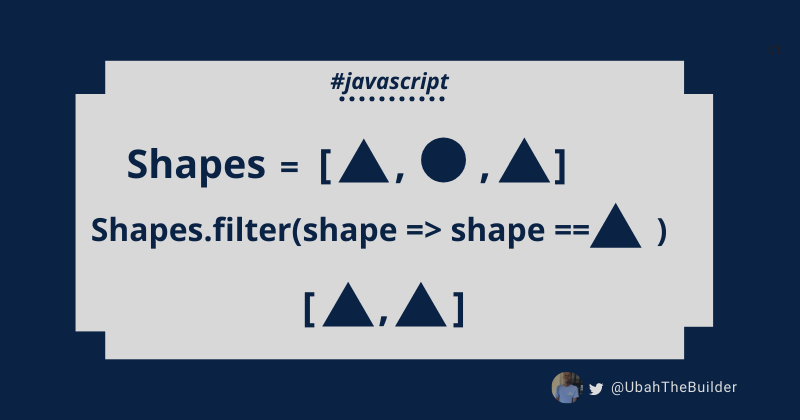
The Array.filter()
method is arguably the most of import and widely used method for iterating over an array in JavaScript.
The style the filter()
method works is very simple. It entails filtering out one or more than items (a subset) from a larger collection of items (a superset) based on some condition/preference.
We all exercise this everyday, whether we're reading, choosing friends or our spouse, choosing what movie to watch, and and so on.
The JavaScript Array.filter()
Method
The filter()
method takes in a callback office and calls that function for every detail it iterates over within the target assortment. The callback part can accept in the following parameters:
-
currentItem
: This is the element in the array which is currently being iterated over. -
alphabetize
: This is the index position of thecurrentItem
inside the array. -
array
: This represents the target assortment along with all its items.
The filter method creates a new array and returns all of the items which pass the condition specified in the callback.
How to Employ the filter()
Method in JavaScript
In the following examples, I will demonstrate how you can use the filter()
method to filter items from an array in JavaScript.
filter()
Case i: How to filter items out of an array
In this example, we filter out every person who is a toddler (whose age falls betwixt 0 and four ).
allow people = [ {name: "aaron",age: 65}, {proper name: "beth",age: 2}, {name: "cara",historic period: 13}, {name: "daniel",age: 3}, {name: "ella",historic period: 25}, {name: "fin",age: 1}, {name: "george",age: 43}, ] let toddlers = people.filter(person => person.age <= 3) console.log(toddlers) /* [{ age: two, name: "beth" }, { age: iii, name: "daniel" }, { age: one, name: "fin" }] */
filter()
Example two: How to filter out items based on a particular property
In this instance, nosotros will only be filtering out the team members who are developers.
let team = [ { name: "aaron", position: "developer" }, { name: "beth", position: "ui designer" }, { name: "cara", position: "programmer" }, { name: "daniel", position: "content director" }, { proper noun: "ella", position: "cto" }, { name: "fin", position: "backend engineer" }, { proper name: "george", position: "developer" }, ] allow developers = squad.filter(member => fellow member.position == "developer") console.log(developers) /* [{ name: "aaron", position: "developer" }, { proper noun: "cara", position: "developer" }, { name: "george", position: "developer" }] */
In the above example, we filtered out the developers. But what if we want to filter out every member who is non a developer instead?
We could practise this:
permit squad = [ { name: "aaron", position: "programmer" }, { name: "beth", position: "ui designer" }, { name: "cara", position: "developer" }, { proper noun: "daniel", position: "content manager" }, { proper noun: "ella", position: "cto" }, { name: "fin", position: "backend engineer" }, { proper name: "george", position: "developer" }, ] permit nondevelopers = team.filter(member => member.position !== "programmer") console.log(nondevelopers) /* [ { name: "beth", position: "ui designer" }, { name: "daniel", position: "content manager" }, { name: "ella", position: "cto" }, { name: "fin", position: "backend engineer" } ] */
filter()
Example 3: How to access the index property
This is a competition. In this competition, at that place are iii winners. The starting time volition get the gold medal, the 2d volition get silver, and the third, statuary.
By using filter
and accessing the index
holding of every item on each iteration, we can filter out each of the 3 winners into different variables.
let winners = ["Anna", "Beth", "Cara"] let gold = winners.filter((winner, alphabetize) => index == 0) permit silver = winners.filter((winner, alphabetize) => index == 1) let bronze = winners.filter((winner, index) => index == ii) panel.log(Gold winner: ${gold}, Silvery Winner: ${argent}, Bronze Winner: ${bronze}) // "Gold winner: Anna, Argent Winner: Beth, Bronze Winner: Cara"
filter()
Example four: How to utilise the array parameter
One of the most mutual uses of the 3rd parameter (array) is to inspect the state of the array being iterated over. For example, nosotros tin can check to see if in that location is another item left in the array. Depending on the outcome, nosotros tin can specify that different things should happen.
In this example, we are going to define an assortment of four people. Even so, since there can just exist 3 winners, the quaternary person in the list will have to be discounted.
To be able to do this, nosotros need to get information about the target array on every iteration.
permit competitors = ["Anna", "Beth", "Cara", "David"] role displayWinners(name, alphabetize, array) { permit isNextItem = index + one < array.length ? true : false if (isNextItem) { console.log(`The No${index+i} winner is ${name}.`); } else { panel.log(`Sorry, ${name} is non one of the winners.`) } } competitors.filter((proper noun, index, array) => displayWinners(proper name, index, assortment)) /* "The No1 winner is Anna." "The No2 winner is Beth." "The No3 winner is Cara." "Lamentable, David is not one of the winners." */

How to Utilize the Context Object
In addition to the callback function, the filter()
method can likewise have in a context object.
filter(callbackfn, contextobj)
This object can and then be referred to from inside the callback role using the this
keyword reference.
filter()
Example five: How to access the context object with this
This is going to be similar to case 1
. We are going to be filtering out every person who falls between the ages of 13 and 19 (teenagers).
Notwithstanding, we will not be hardcoding the values within of the callback function. Instead, we will define these values 13 and 19 as properties inside the range
object, which we will subsequently pass intofilter
as the context object (second parameter).
allow people = [ {proper name: "aaron", age: 65}, {name: "beth", historic period: 15}, {name: "cara", age: xiii}, {name: "daniel", historic period: 3}, {proper name: "ella", age: 25}, {name: "fin", age: 16}, {name: "george", age: eighteen}, ] let range = { lower: 13, upper: 16 } let teenagers = people.filter(function(person) { return person.age >= this.lower && person.age <= this.upper; }, range) console.log(teenagers) /* [{ age: 15, name: "beth" }, { historic period: 13, proper noun: "cara" }, { age: 16, proper noun: "fin" }] */
We passed the range
object as a second statement to filter()
. At that point, it became our context object. Consequently, nosotros were able to access our upper and lower ranges in our callback function with the this.upper
and this.lower
reference respectively.
Wrapping Upwardly
The filter()
array method filters out particular(s) which match the callback expression and returns them.
In addition to the callback part, the filter
method tin besides take in a context object as the second argument. This will enable you access any of its properties from the callback function using this
.
I hope y'all got something useful from this article.
I f you want to learn more than about Web Evolution, feel free to visit my Blog .
Thank you for reading and see you lot presently.
P/S : If yous are learning JavaScript, I created an eBook which teaches 50 topics in JavaScript with mitt-drawn digital notes. Check it out here.
Larn to code for free. freeCodeCamp's open up source curriculum has helped more than 40,000 people go jobs every bit developers. Go started
How To Use Filter To Only Grab Objects That Contain A Certain String From An Array,
Source: https://www.freecodecamp.org/news/javascript-array-filter-tutorial-how-to-iterate-through-elements-in-an-array/
Posted by: stewartfaturaved.blogspot.com
0 Response to "How To Use Filter To Only Grab Objects That Contain A Certain String From An Array"
Post a Comment